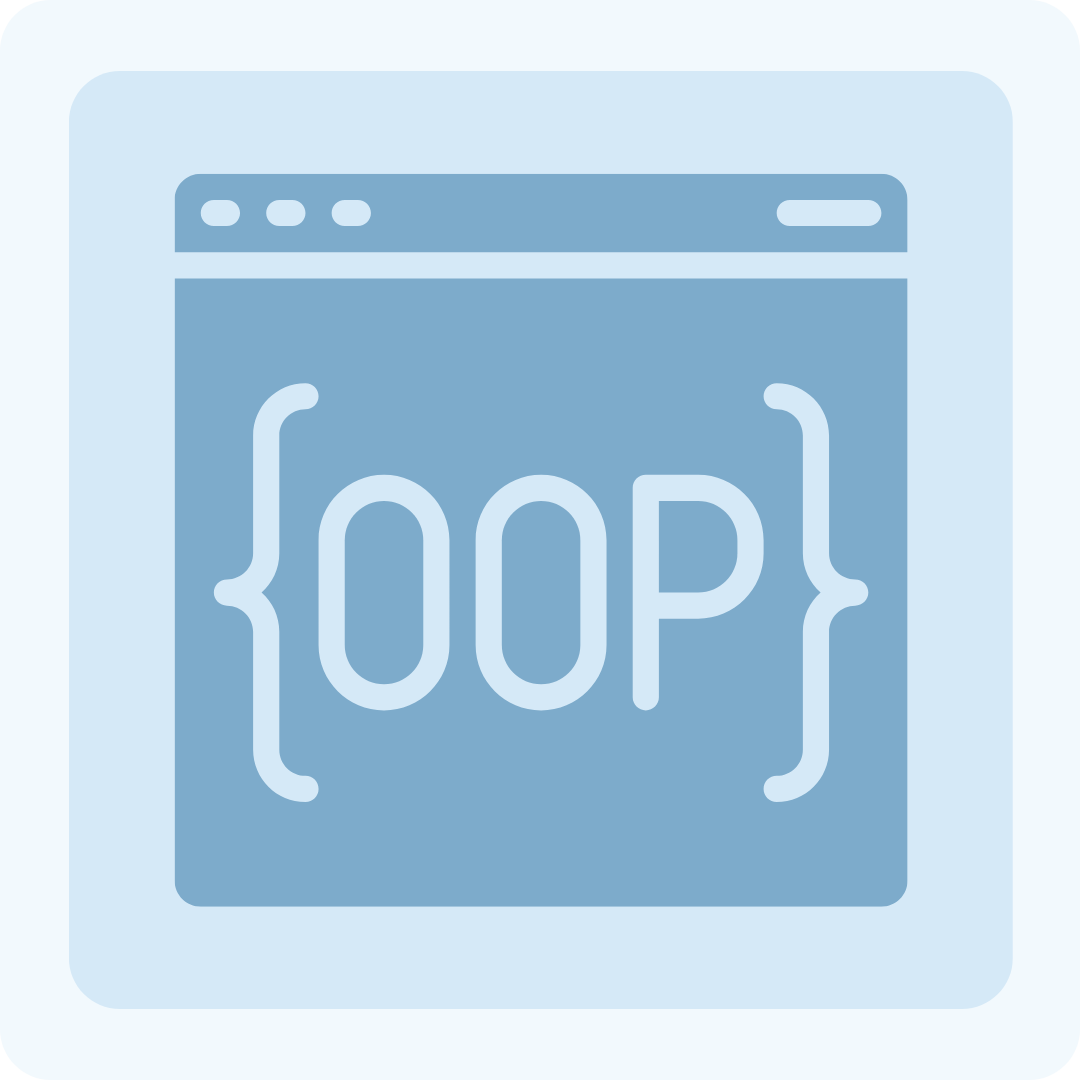
Greedy Algorithms
Concept
Select the best option at the moment, without concern for the future (only leads to optimal solutions for certain types of problems)
Implementation
Very varied, but often includes simple logic that evaluates a situation and makes an optimal decision at that point
Video Explanation
Example Problems
Recursion
Concept
An algorithm that breaks itself into sub-problems and repeats on those smaller instances
Implementation
A function that has base cases, breaks the input into smaller pieces, calls itself on those pieces, and (maybe) does something with the results
Video Explanation
Example Problems
Dynamic Programming
Concept
Recursive algorithms that save information to prevent repeated work
Implementation
Same as recursion, except with an additional list, matrix, or dictionary that records the results of completed function calls
Video Explanation
Example Problems
Bit Manipulation
Concept
Algorithmically move or change the bits of some data to produce a desired result
Implementation
Often involves operations like NOT, AND, OR, XOR, and left/right shifts
Video Explanation
Example Problems
Object Oriented Programming (OOP)
Concept
A programming style that organizes software around objects rather than functions
Implementation
Uses classes and subclasses, private variables, object instantiation, etc.
Four Pillars
Abstraction, Polymorphism, Inheritance, Encapsulation (APIE)
Video Explanation
Short-Circuit EvaluationConcept
Instances when the compiler skips the execution of certain sub-expressions in code logic as soon as the value is determined
Implementation
Very language-dependent, look for answers specific to a language online
Example (Python)
If a variable var is None, then only the first half of the expression “if var and var == 7” will be run
Video Explanation
Garbage CollectionConcept
Memory management to ensure that a program doesn’t use infinite memory
Implementation
Very language-dependent, (for example, it is handled by default in Python but must be handled by the user in C)
Example (C)
The user must “free” any memory they “allocate”
Video Explanation
Mutable vs. Immutable
Concept
Certain variables can be changed after being created, while others must be re-created if they need to be changes
Implementation
Very language-dependent
Example (Python)
Strings and integers are immutable, lists and dictionaries are mutable
Video Explanation
System DesignAlmost no internship interviews will ask system design questions. However, a company tells you to prepare specifically for this type of question, we recommend working from this cheat sheet.
Agile Development and ScrumAgile Development is a project management methodology that many software teams use to organize their work. The most common Agile framework is “Scrum,” which involves organizing work into “sprints'' (usually 2-week periods). Scrum uses various tools to plan work, assign tasks, and assess past performance. The framework also emphasizes communication between team members. These concepts are unlikely to come up during interviews, but you should be familiar with them just in case.
General Tips
- For Array problems, try a Hashtable
- For Palindrome problems, try a Stack
- For Linked Lists, try fast and slow pointers
- For Breadth First Search, use a Queue
- For Depth First Search, use a Stack
- If a function doesn’t have the arguments you’d prefer, use a helper function with whatever arguments you want